What Is Python (An Overview)
Python is a programming language that was created in the 1980s. It is widely used today because it is easy to read and write and supports multiple platforms. Python works for web development, scientific computing, artificial intelligence, and software engineering. It is also one of the most popular languages for teaching computer programming to beginners.
The main reason why most people use Python is that it is a very versatile language that can be used for many different purposes. Additionally, Python has many libraries and frameworks that make it even more powerful. One of the most important ones is Python's indentation rules. For example, the if statement:
if age<21: print "You cannot buy wine ! \n" print "But you can buy chewing gum. \n"print "this is outside if\n" |
There are many other essential statements and commands. What we are going to talk about today is the way to delete a file in Python. Read the methods below and clear Python files with simple steps.
How to Delete a File in Python (3 Ways)
Python gives you several ways to delete files. You can use the OS module, the Shutil module, or the Pathlib module. Each module has its advantages and disadvantages, so it's important to choose the right one for your needs to force delete file.
Method 1. How to Delete a File with the OS Module in Python
The OS module is the most basic way to delete files in Python. It offers a straightforward interface that can be used to delete single files or entire directories. However, it doesn't offer any features for managing permissions or error handling. To delete a file using the OS module:
import os file_path = if os.path.isfile(file_path): os.remove(file_path) print("File does not exist") |
Method 2. How to Delete a File with the Shutil Module in Python
The Shutil module is a more comprehensive tool for deleting files in Python. It can delete single files, directories, and even entire directory trees. It also offers some features for managing permissions and error handling. However, it can be somewhat complex, so it's important to read the documentation carefully before using it.
Follow the steps below to Use Python to delete a file if it exists.
import shutil shutil.rmtree('path') |
Now here is the place of the path you can provide the path (e.g. /home/school/math/final) to remove existing files.
Method 3. How to Delete a File with the Pathlib Module in Python
The Pathlib module is a newer addition to Python that offers an object-oriented interface for working with files and directories. It's very easy to use and provides helpful features, such as the ability to delete multiple files simultaneously. However, it doesn't offer any support for permissions or error handling.
You can watch this video first to see the Python deleting process with details.
- 0:01 Introduction
- 0:36 Import files
- 1:29 Delete files in Python
You can use the following code to clear/delete the file or folder:
Step 1. Create a Path object.
import pathlib p_object = Path(".") type(p_object) |
Step 2. Use the unlink() function to delete a file.
import pathlib file = pathlib.Path("test/file.txt") file.unlink() |
Step 3. use the rmdir() function to delete the directory.
import pathlib directory = pathlib.Path("files/") directory.rmdir() |
If you still have problems on how to choose the right code, you can check the comparison table below to get additional help.
Situations | Command |
---|---|
Delete a single file |
|
Delete/empty directories? | rmdir() |
Delete non-empty directories | rmtree() |
Python Data Recovery: How to Recover a File Deleted with Python
Can you get it back when it comes to an accidentally deleted file? The answer is yes. There are two ways to recover Python deleted data. One uses the restore feature in Python, and the other uses reliable data recovery software.
Tip 1. Recover Deleted Files with Python
Python deleted files can be recovered if you find your history folder in Python. Here are the steps to recover Python deleted files.
Step 1. Right-click on the folder above the deleted file and select "Local History > Show History".
Step 2. Select the wanted file or folder and click "revert" on the top left.
Tip 2. Run Python Data Recovery with Data Recovery Software
The second way to recover deleted files is using data recovery software. Python data recovery software is designed to search through all the drives on your computer for any traces of Python files and then recover them. These programs are very effective and can help you recover your deleted files quickly and easily. One of the most effective file recovery tools is EaseUS Data Recovery Wizard.
EaseUS free data recovery software is a powerful data recovery software that can be used to recover deleted files and data from Python databases. The software offers a wide range of features for deleted file recovery.
- Support various file formats, such as jpg, png, bmp, and gif.
- Restore lost data due to partition loss or damage, software crash, virus infection, and other unexpected events.
- Recover deleted files from internal and external hard drives, including FAT, NTFS, and exFAT file systems.
Overall, EaseUS Data Recovery Wizard is an excellent choice for recovering files and data deleted by Python. Follow the steps below to recover deleted Python data.
Step 1. Choose the exact file location and then click the "Scan" button to continue.
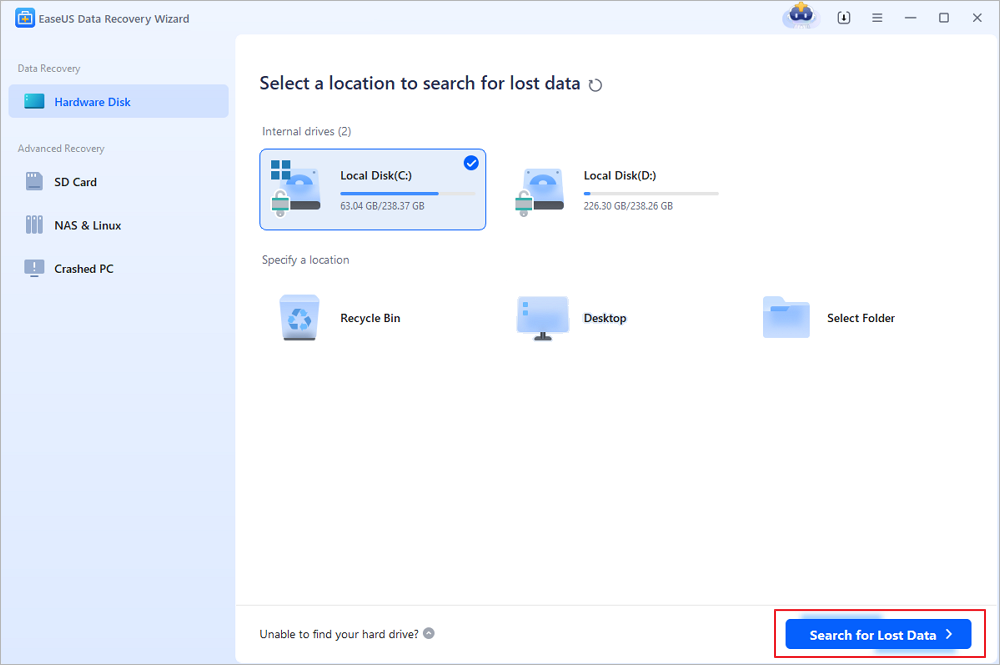
Step 2. After the process, select the "Deleted Files" and "Other Lost Files" folders in the left panel. Then, you can apply the "Filter" feature or click the "Search files or folders" button to find the deleted files.
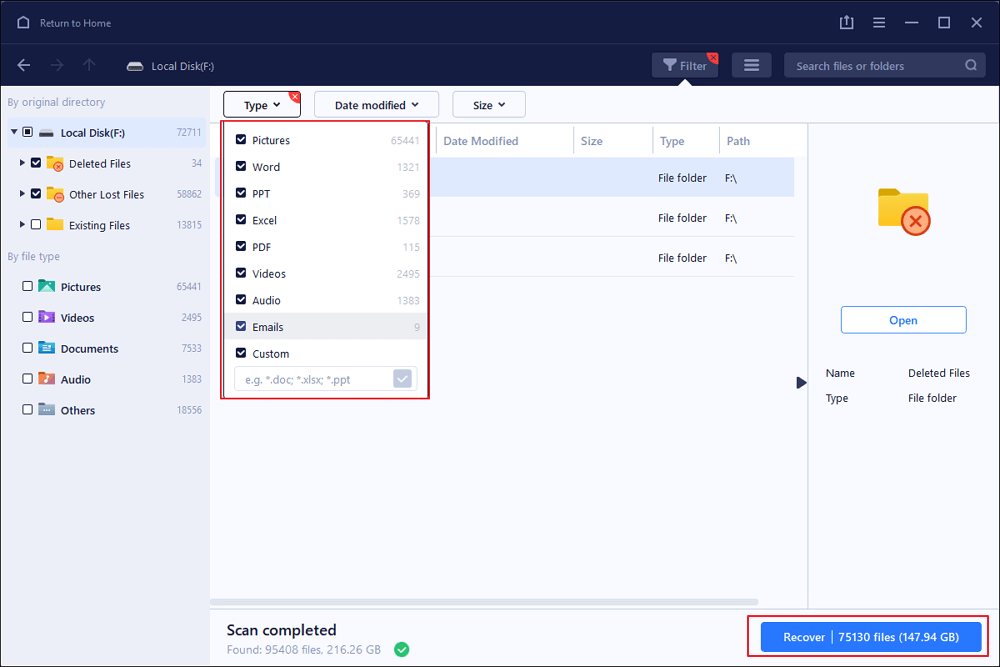
Step 3. Click the "Recover" button and save the restored files – ideally, it should be different from the original one.
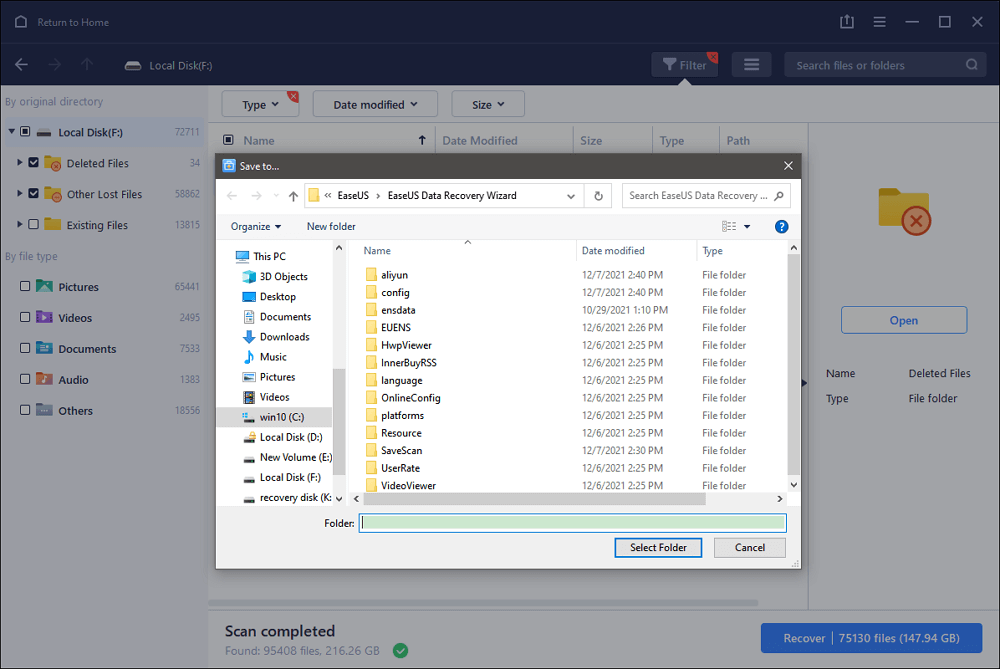
Conclusion
In conclusion, Python has a few different ways to delete a file. The most straightforward way is to use the built-in "os" module. This module provides many functions for interacting with the operating system. If you want to delete a directory and all its contents, you can use the "Shutil" module. The "Shutil" module provides high-level operations on files and directories. Finally, if you want to delete an open file, you can use the "os.unlink" function. This function deletes a single file, regardless of whether it is open or not.
If Python deletes a file you don't want to delete, you can recover Python data with the built-in "revert" option or data recovery software.
Python Delete Files FAQs
To get additional help, read the Python-related questions and answers below.
1. How do I delete a text file in Python?
Use file. truncate() to erase the file contents of a text file
- file = open(sample.txt, r+)
- file. truncate(0)
- file. close()
2. How to fix Python Setup.py egg_info?
To fix Python setup.py egg_info:
- Check if Pip and Setuptools Are Installed Correctly
- Upgrade Pip to Fix Python Setup.py egg_info
- Upgrade Setuptools
- Try to Install the ez_setup
3. How do you delete a file if it already exists in Python?
There are three ways to remove a file if it exists and handle errors:
- Run os. remove
- Use shutil module
- Run os. unlink
Was This Page Helpful?

Daisy is the Senior editor of the writing team for EaseUS. She has been working at EaseUS for over ten years, starting as a technical writer and moving on to being a team leader of the content group. As a professional author for over ten years, she writes a lot to help people overcome their tech troubles.

Brithny is a technology enthusiast, aiming to make readers' tech lives easy and enjoyable. She loves exploring new technologies and writing technical how-to tips. In her spare time, she loves sharing things about her game experience on Facebook or Twitter.
Related Articles
-
How to Fix the Recovery Server Could Not Be Contacted on macOS Sonoma/Ventura
Brithny/2024-01-11
-
Desktop Icons Not Showing Properly on Windows 10/11
Larissa/2024-03-19
-
How to Fix Diskpart Failed to Clear Disk Attributes Error
Brithny/2024-01-11
-
How to Export Chrome Bookmarks to Safari - 2024 Updated
Larissa/2024-01-11
EaseUS Data Recovery Services
EaseUS data recovery experts have uneaqualed expertise to repair disks/systems and salvage data from all devices like RAID, HDD, SSD, USB, etc.